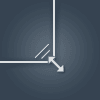
Also available in: Français
If you are a frequently user of JS, you have certainly noticed that some events are triggered quite occasionally, and others can be triggered very frequently and become quite difficult to manage, as in the case of “onscroll” and “onresize” for example.
When you implement an event listener on one of these events (I’ll take onresize
for the following examples) you’ll quickly see when resizing the window that they are executed each time the pixel changes within the window width.
The code used most of the time
Often, and quite simply, we implement a listener like this:
window.addEventListener('resize', function(){
console.log('resizing');
});
If you add this to the JS scripts of your project, and open the page on your browser, you will notice when you open your console (F12) and resize the window, that the console panics a little.
Imagine that instead of a console.log()
your script checks the DOM, changes the structure under certain conditions (screen width, visibility of an element, for example), etc., and this for each pixel of the resizing….
Don’t laugh, we agree that resizing the window like this is not common for the user, but it was (or still is) a client’s method to test if the responsive was working well, and he thought it was a little slow on his Mac. I don’t blame him, he probably read somewhere that the responsive is when you resize your browser window.
In short, we can optimize the execution frequency of all this code when resizing, let’s see that.
The same function, but a bit different
The principle is to create a timeout
that is run at resizing and destroyed at resizing. Yeah, I know, it’s weird, but you’ll understand.
var the_timer;
window.addEventListener('resize', function(){
clearTimeout(the_timer);
the_timer = setTimeout(function(){
console.log('resized');
}, 75);
});
To give you a short explanation, just read the code in a linear way: when resizing, we delete the timeout
(this stops the execution of the function as a parameter), then we launch the timeout
. This has an execution delay of 75 milliseconds. So, if less than 75 ms have elapsed between the first resize
trigger and the second, the timeout
is reset even before the function is executed in the first parameter. Did you follow me?
What makes it work is the (global) the_timer
variable declared before the listener.
There you go, it’s a little better though. The frequency, or “sensitivity” is set to the number of milliseconds (here
75
) entered for the timeout
. The higher this number, the faster the content of your function will be executed.
This method is not necessarily adapted to all cases for all projects, especially in the context of the onscroll
event, so measure the impact of this implementation well 🙂
I hope this tip will be useful to you.
Post a comment for this article?
Follow comments and trackbacks