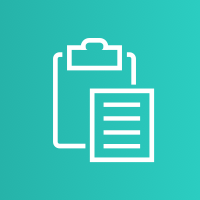
Also available in: Français
You certainly already met those buttons to “Copy into Clipboard”. They usually use a trick made with Flash to overcome a security feature provided by our web browsers? Avec the evolution of JS API and the listening of developer requests, you can now (since some months) do it with JavaScript natively.
The technic is quite simple. You just need to use the execCommand()
function available at the document
level.
What is execCommand()
The execCommand()
function looks like that:
where the parameters are:
commandName
:
the command name to execute (copy
,paste
,undo
,redo
,insertHTML
, etc.)showDefaultUI
:
true
orfalse
, to use the default interface (or not… not supported bu Mozilla at the moment)valueArgument
:
some commands need an argument, for example theinsertImage
command which is waiting for an URL as value.
As its name tells us, it provide to you the ability to execute a command inside the current window. To get a better and complete documentation the MDN article is a good resource.
Code snippet
To give you the essential point of this feature, I will write a minimalist code example. I’ll let you click on the demo link below (which presents a code too) if you want a more concret how-to-use example.
Let’s imagine this HTML:
The next JavaScript code is very simple and provides you the main “copy in your clipboard” function. The content of the textarea element will be copied on a simple button click. Then, you can paste this content where ever you want. (other input field, editing document, etc.)
When document.execCommand()
succeeded it returns true
, at the opposite if something went wrong (no support or error) it returns false
.
I let you imagine what you can do with that. By the way, take a look at this example:
Share your code on CodePen and in a new comment in that blog to show your results, I’m curious 🙂
Have fun!
Post a comment for this article?
Follow comments and trackbacks